Organization API Credentials
Create OAuth 2 clients to access organization, project, and client management APIs.
This guide explains how to manage and use OAuth 2 clients to access organization-level management APIs. It outlines how to use the Client Credentials grant to authorize API requests and provides examples for working with the management APIs. OAuth 2 clients allow you to dynamically grant permissions and automate access control to Pangea management APIs.
On the Organization Settings page (accessible from the link under your profile icon in the Pangea User Console), go to the Organization API Credentials page to manage access to management APIs within your organization.
Management Clients
Organization-level OAuth 2 management clients can issue access tokens using the Client Credentials grant. These tokens can be used to authorize access to the following APIs:
- Service & Management Client APIs - Configure and manage OAuth 2 clients in the organization.
- Platform Organization APIs - Manage organization details.
- Platform Project APIs - Manage projects within the organization.
Create management client
-
Click the Create management client button.
-
In the Create a client dialog, configure the client:
-
Name - Enter a name that will appear in the Client Name column in the client list.
-
Platform Client secret rotates every - Specify how often the client secret is rotated in Vault.
-
Access tokens expire in - Set the lifetime of access tokens issued by the client.
-
Organization Role - Define the specific role (such as Organization Admin) assigned to the identity represented by the client's access tokens within the organization context. This role grants access to organization-level resources. Access to API routes is further controlled by the client's scope.
-
Complete API access - Select the scope values that the client can request in its access tokens to access the corresponding management API endpoints.
-
pangea:platform:account:read
pangea:platform:account:manage
Access to the Service & Management Client APIs .
-
pangea:platform:org:read
pangea:platform:org:manage
Access to the Platform Organization APIs .
-
pangea:platform:project:read
pangea:platform:project:manage
Access to the Platform Project APIs .
-
-
-
Click Create client.
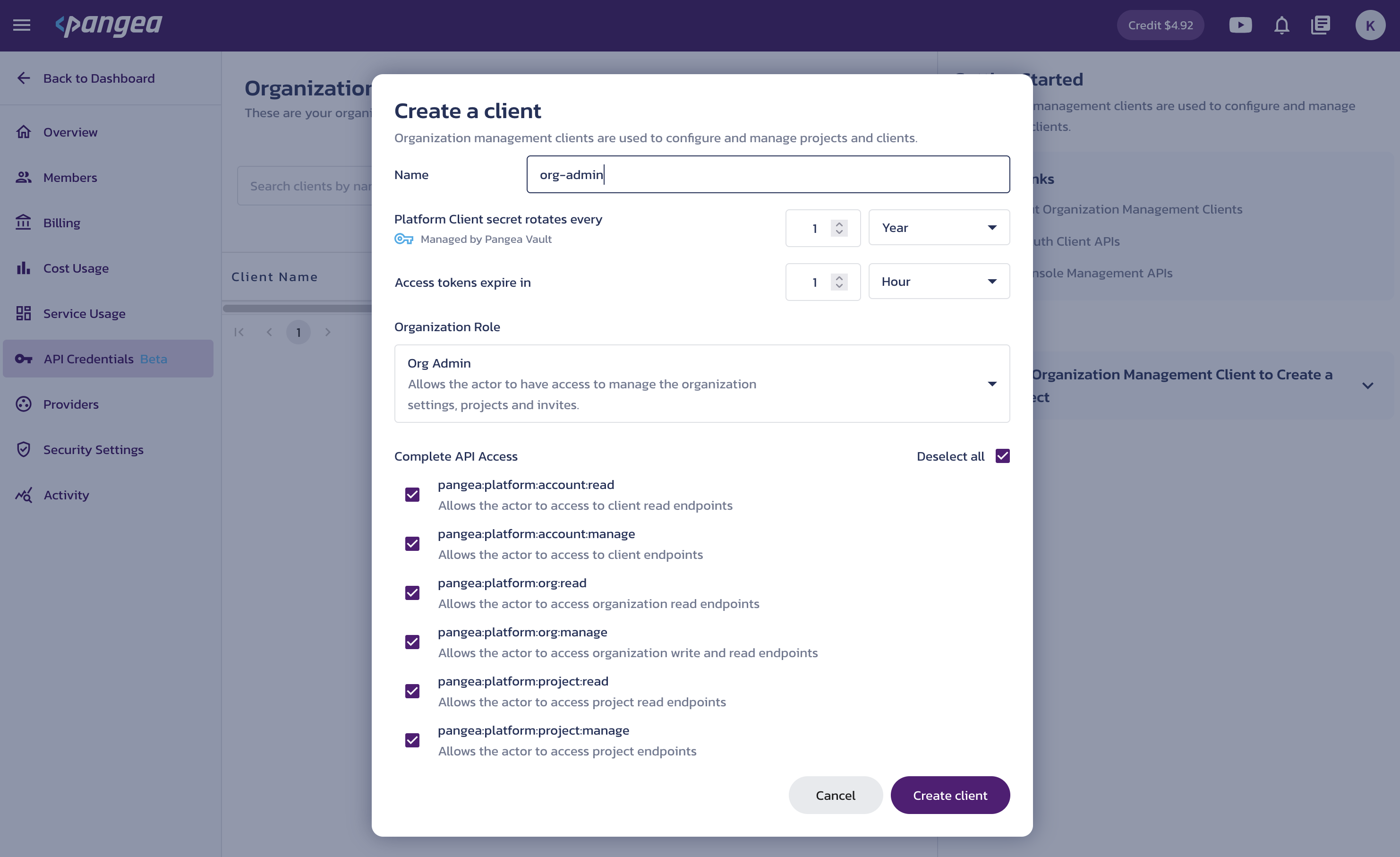
Client details
The new client ID and secret, along with the Create access token button, are shown in a temporary view. Once closed, this view cannot be reopened. However, you can:
- Use the client list table to copy the client ID and secret and view other client details.
- Use the key link to configure the client secret rotation policy in Vault.
- Use the triple-dot menu to create a new access token, generate a new client secret, or delete the client.
- Click a client row to view its details in the right-hand panel, update its configuration, or create new secrets.
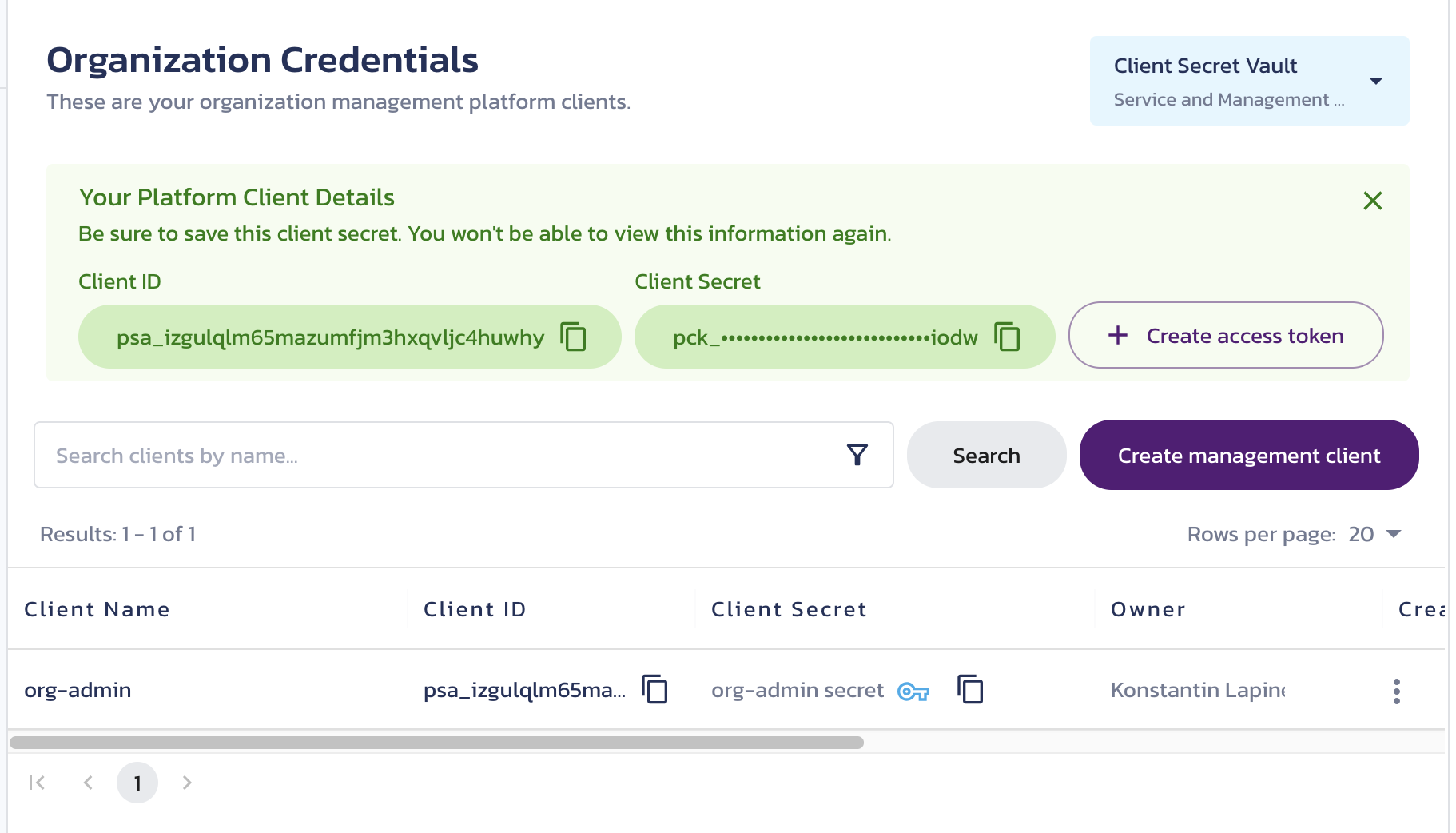
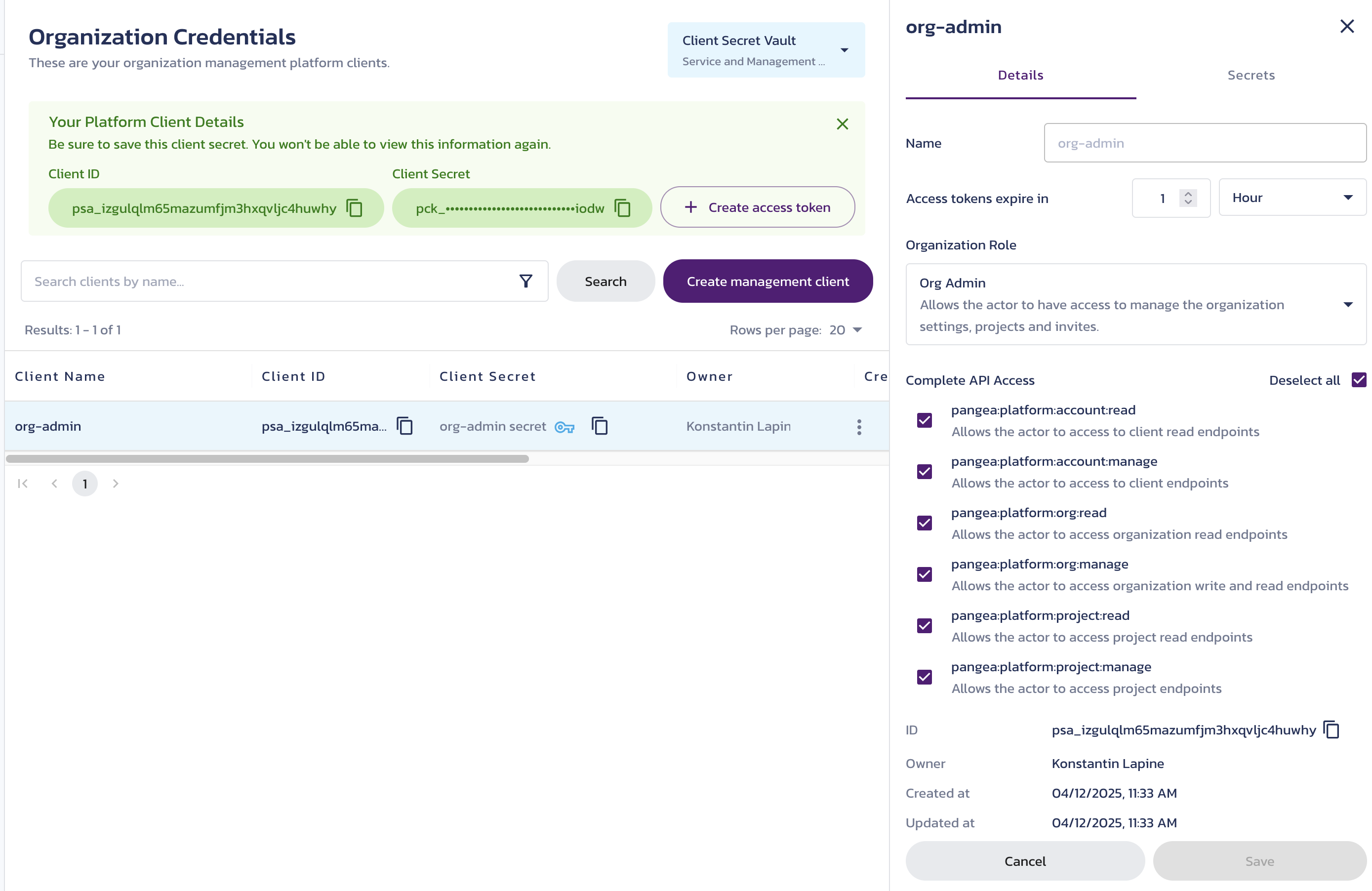
Client Secret Vault
Client secrets are stored in Vault under the project selected in the Client Secret Vault dropdown in the upper right. You can only access secrets stored in the Vault of the currently selected project.
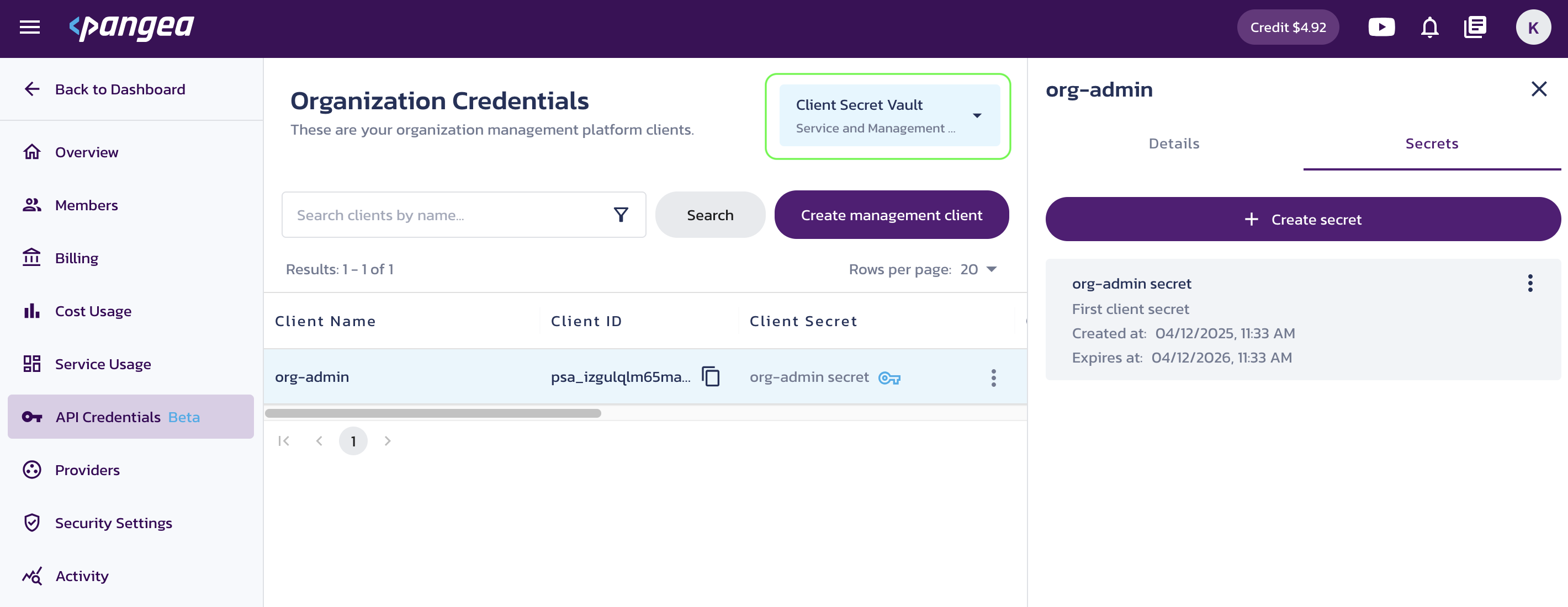
Management APIs
Client Credentials grant
Your application can use the endpoints returned by the Service & Management Clients' OAuth Authorization Server Metadata endpoint to obtain access tokens using the Client Credentials grant and to revoke authorization.
curl --location 'https://authorization.access.aws.us.pangea.cloud/.well-known/oauth-authorization-server'
{
"grant_types_supported": [
"client_credentials"
],
"introspection_endpoint": "https://authorization.access.aws.us.pangea.cloud/v1beta/oauth/token/introspect",
"issuer": "https://authorization.access.aws.us.pangea.cloud",
"response_types_supported": [
"token"
],
"revocation_endpoint": "https://authorization.access.aws.us.pangea.cloud/v1beta/oauth/token/revoke",
"scopes_supported": [
"pangea:platform:account:read",
"pangea:platform:account:write",
...
"pangea:service:vault:sign",
"pangea:service:vault:config:manage",
"pangea:service:vault:config:read"
],
"token_endpoint": "https://authorization.access.aws.us.pangea.cloud/v1beta/oauth/token",
"token_endpoint_auth_methods_supported": [
"client_secret_basic",
"client_secret_post"
]
}
Authorize client requests
By default, a new client is registered with the client_secret_basic
authentication method.
You can use the Service & Management Clients APIs to register a Platform Client with the client_secret_post
authentication method instead.
In the following example, we'll use the default HTTP Basic Authentication scheme to authenticate the client.
-
Set the environment.
Set environment variablesexport PANGEA_CLIENT_ID="psa_hd5cnx3sh64jrz3ruu6r4t4jsk2zj6nn"
export PANGEA_CLIENT_SECRET="pck_65mjv4...imykaf" -
Concatenate the client ID and client secret with a colon (
:
) and base64 encode the result.tipOn a Linux-based system, you can use the
base64
utility to encode the client credentials:Use HTTP Basic authentication scheme for authenticating a clientexport PANGEA_BASIC_AUTHENTICATION_CREDENTIAL=$(echo -n $PANGEA_CLIENT_ID:$PANGEA_CLIENT_SECRET | base64)
-
Add the Base64-encoded string to the request's Authorization header, prefixed with "Basic ".
-
Provide the following parameters in the "application/x-www-form-urlencoded" format:
-
grant_type
- Set to "client_credentials". -
scope
(optional) - A space-delimited list of scope values defining which endpoints the token can access.If you include the
scope
parameter, the token will be limited to the specified subset of client permissions. Ifscope
is omitted, the token will inherit all permissions granted to the client.
-
Request access token
export PANGEA_TOKEN_ENDPOINT="https://authorization.access.aws.us.pangea.cloud/v1beta/oauth/token"
curl --location "$PANGEA_TOKEN_ENDPOINT" \
--header "Authorization: Basic $PANGEA_BASIC_AUTHENTICATION_CREDENTIAL" \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode 'grant_type=client_credentials' \
--data-urlencode 'scope=pangea:platform:org:read pangea:platform:project:read pangea:platform:project:manage pangea:platform:account:read pangea:platform:account:manage'
The response includes the access token, its expiration time, and the scope granted to the token.
{
"access_token": "pts_ivfc3u...o3r66",
"token_type": "Bearer",
"expires_in": 3599,
"scope": "pangea:platform:project:manage pangea:platform:account:read pangea:platform:account:manage pangea:platform:org:read pangea:platform:project:read"
}
Introspect access token
Optionally, you can verify whether the access token is active and check its scope before making an API request.
export PANGEA_INTROSPECTION_ENDPOINT="https://authorization.access.aws.us.pangea.cloud/v1beta/oauth/token/introspect"
curl --location "$PANGEA_INTROSPECTION_ENDPOINT" \
--header "Authorization: Basic $PANGEA_BASIC_AUTHENTICATION_CREDENTIAL" \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode "token=$PANGEA_ACCESS_TOKEN"
A properly authorized introspection request for an active token returns token information with the active
key set to true
.
{
"iss": "https://authorization.access.aws.us.pangea.cloud",
"sub": "pui_55ljz3kllliqrl43pxdgho4bk62qibzk",
"exp": 1745192332,
"nbf": 1745188732,
"iat": 1745188732,
"jti": "pmt_oa22hawfpbpnncpjizapny4qkge7z2bh",
"client_id": "psa_izgulqlm65mazumfjm3hxqvljc4huwhy",
"token_type": "Bearer",
"username": "org-admin",
"scope": "pangea:platform:project:manage pangea:platform:account:read pangea:platform:account:manage pangea:platform:org:read pangea:platform:project:read",
"active": true
}
If the token is inactive, invalid, or the request is unauthorized, the response contains only the active
key set to false
.
{
"active": false
}
Revoke access
export PANGEA_REVOCATION_ENDPOINT="https://authorization.access.aws.us.pangea.cloud/v1beta/oauth/token/revoke"
curl --location "$PANGEA_REVOCATION_ENDPOINT" \
--header "Authorization: Basic $PANGEA_BASIC_AUTHENTICATION_CREDENTIAL" \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data-urlencode "token=$PANGEA_ACCESS_TOKEN"
A successful revocation request returns HTTP status code 200
with no response body.
If the request succeeds but revocation fails, the response body will include details about the error.
{
"error": "invalid_request",
"error_description": "The token does not exist"
}
Service & Management Clients APIs
Once you obtain an access token using the Client Credentials grant issued by a management client, and the token has access to the Service & Management Clients APIs, you can make it available to your application and use it to authorize requests by passing it as a bearer token.
export PANGEA_DOMAIN="aws.us.pangea.cloud"
export PANGEA_ACCESS_TOKEN="pts_mxui3w...wo7z56"
Register an Organization Management Client
Register a client using the /v1beta/oauth/clients/register endpoint.
You must use an organization management client to authorize the registration of another client with organization-level permissions.
Parameters
-
scope
- Provide space-delimited scope values that define which management API endpoints the application client can access in its access tokens.-
pangea:platform:account:read
pangea:platform:account:manage
Access to the Service & Management Client APIs .
-
pangea:platform:org:read
pangea:platform:org:manage
Access to the Platform Organization APIs .
-
pangea:platform:project:read
pangea:platform:project:manage
Access to the Platform Project APIs .
-
Optionally, provide:
-
roles
- An array of roles to assign to the client.Role assignment is optional during registration and can be completed later using the /v1beta/oauth/clients/{client_id}/grant endpoint.
Without a role assigned, the client cannot authorize access to any endpoints. The organization-level management client can be assigned a single role granting access to organization resources:
type
- Type of entity to which access is granted. Set to "organization".id
- The organization ID.role
- Level of access. Set to "admin".
client_name
- Specify the client name as it will appear in the Pangea User Console. For example, use it to indicate which application is using the client.token_endpoint_auth_method
- Choose the authentication method for the client:client_secret_basic
(default) - Sends client credentials in theAuthorization
header using theBasic
scheme. See Authorize client requests.client_secret_post
- Sends client credentials in the request body usingclient_id
andclient_secret
.
client_secret_expires_in
- Specify how often the client secret is rotated in Vault.client_secret_name
- Name the automatically created client secret.client_secret_description
- Add a description for the secret, as shown on the secret details page in the Pangea User Console.access_token_expires_in
- Set the lifetime of access tokens issued by the client.
Create client
The following example demonstrates how to register an organization admin client with access to the Service & Management Client APIs , which can be used to dynamically register additional clients.
For example, you can use this client to programmatically create other organization-level clients or project management clients for managing projects and services within your organization.
export PANGEA_ORGANIZATION_ID="poi_qie5kaj5o3622tw4qj5qbyw77ship4bh"
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/register" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"client_name": "My Organization Admin Client",
"scope": "pangea:platform:account:read pangea:platform:account:manage pangea:platform:project:read pangea:platform:project:manage",
"roles": [
{
"id": "'"$PANGEA_ORGANIZATION_ID"'",
"type": "organization",
"role": "admin"
}
]
}'
The response will include the newly created client information. The client will also appear on the Organization API Credentials page in your Pangea User Console .
{
"client_id": "psa_xvjxu3if5tquh3ffahtdrf44vvyjbbkl",
"created_at": "2025-04-19T01:42:16.989672Z",
"updated_at": "2025-04-19T01:42:16.989672Z",
"client_name": "My Organization Admin Client",
"scope": "pangea:platform:account:read pangea:platform:account:manage pangea:platform:project:read pangea:platform:project:manage",
"token_endpoint_auth_method": "client_secret_basic",
"redirect_uris": [],
"grant_types": [
"client_credentials"
],
"response_types": [
"token"
],
"client_token_expires_in": 3600,
"client_secret_id": "pce_ubljg5pddwrzd6csxi7irbacffhn4sdo",
"client_secret": "pck_cxvejh...oeswdp",
"client_secret_expires_at": "2026-04-19T01:42:16.988847Z",
"client_secret_name": "My Organization Admin Client Secret",
"client_secret_description": "Auto-created first client secret",
"owner_id": "pui_55ljz3kllliqrl43pxdgho4bk62qibzk",
"owner_username": "konstantin.lapine@pangea.cloud",
"creator_id": "psa_izgulqlm65mazumfjm3hxqvljc4huwhy",
"client_class": "management",
"tenanted_by": "organization"
}
The client secret, along with the client ID, is saved and managed in Vault. You can reference the secret by its client_secret_id
and use the Vault APIs to dynamically retrieve or rotate it.
Grant client additional access
You can use the /v1beta/oauth/clients/{client_id}/grant endpoint to extend a client's scope and set roles if this was not done during registration.
Parameters:
roles
- An array of role objects. The organization management client created above does not accept additional roles, so leave the array empty.scope
- A space-delimited list of additional scope values that the client can assign to its access tokens.
The client registered above has access to client and project management APIs within the organization, but not to the organization management APIs. In the following example, we extend the client's scope to allow it to authorize access to organization management APIs.
export PANGEA_CLIENT_ID="psa_xvjxu3if5tquh3ffahtdrf44vvyjbbkl"
export PANGEA_ORGANIZATION_ID="poi_qie5kaj5o3622tw4qj5qbyw77ship4bh"
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID/grant" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"roles": [],
"scope": "pangea:platform:org:read pangea:platform:org:manage"
}'
A successful assignment returns a 200
status code with an empty response body.
Revoke client access
You can (partially) revoke client access using the /v1beta/oauth/clients/{client_id}/revoke endpoint. For example:
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID/revoke" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"roles": [],
"scope": "pangea:platform:org:manage"
}'
A successful revocation returns a 200
status code with an empty response body.
Check client access
The client information returned from the registration endpoint or the /v1beta/oauth/clients/{clientId} endpoint includes the client’s API access, defined by its assigned scope.
export PANGEA_CLIENT_ID="psa_xvjxu3if5tquh3ffahtdrf44vvyjbbkl"
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID" \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN"
{
"client_id": "psa_xvjxu3if5tquh3ffahtdrf44vvyjbbkl",
"scope": "pangea:platform:project:manage pangea:platform:org:read pangea:platform:account:read pangea:platform:account:manage pangea:platform:project:read",
...
}
To check a client's permissions, you can retrieve its roles using the /v1beta/oauth/clients/{clientId}/roles endpoint.
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID/roles" \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN"
{
"roles": [
{
"type": "organization",
"id": "poi_qie5kaj5o3622tw4qj5qbyw77ship4bh",
"service": "",
"role": "admin"
}
],
"last": "MTox",
"count": 1
}
Register a Project Management Client
Register a client using the /v1beta/oauth/clients/register endpoint.
Parameters
-
scope
- Provide space-delimited scope values that define which management API endpoints the application client can access in its access tokens.-
pangea:platform:account:read
pangea:platform:account:manage
Access to the Service & Management Client APIs .
-
pangea:platform:project:read
pangea:platform:project:manage
Access to the Platform Project APIs .
-
pangea:service:audit:config:manage
pangea:service:redact:config:manage
Access to the Service Configuration APIs .
-
Optionally, provide:
-
roles
- An array of roles to assign to the client.Role assignment is optional during registration and can be completed later using the /v1beta/oauth/clients/{client_id}/grant endpoint.
Without a role assigned, the client cannot authorize access to any endpoints. The project-level management client can be assigned a single role granting access to project resources:
type
- Type of entity to which access is granted. Set to "project".id
- The project ID.role
- Level of access. Set to "admin".
client_name
- Specify the client name as it will appear in the Pangea User Console. For example, use it to indicate which application is using the client.token_endpoint_auth_method
- Choose the authentication method for the client:client_secret_basic
(default) - Sends client credentials in theAuthorization
header using theBasic
scheme. See Authorize client requests.client_secret_post
- Sends client credentials in the request body usingclient_id
andclient_secret
.
client_secret_expires_in
- Specify how often the client secret is rotated in Vault.client_secret_name
- Name the automatically created client secret.client_secret_description
- Add a description for the secret, as shown on the secret details page in the Pangea User Console.access_token_expires_in
- Set the lifetime of access tokens issued by the client.
Create client
The following example demonstrates how to register a Project Admin client with access to the Service & Management Client APIs . This client can be used to dynamically register additional clients.
For example, you can use it with the Service & Management Clients APIs to programmatically create service clients for consuming Pangea security services - per project, customer, or pipeline.
export PANGEA_PROJECT_ID="ppi_vjmsx2q26gkqaxa2eit6karm2ccdbk7s"
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/register" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"client_name": "My Project Admin Client",
"scope": "pangea:platform:account:read pangea:platform:account:manage",
"roles": [
{
"id": "'"$PANGEA_PROJECT_ID"'",
"type": "project",
"role": "admin"
}
]
}'
The response will include the newly created client information. The client will also appear under the Management Clients tab on the Project API Credentials page in your Pangea User Console .
{
"client_id": "psa_kzz2pmojcpru524txqz5ilkrnzlkbcot",
"created_at": "2025-04-16T23:43:00.755990Z",
"updated_at": "2025-04-16T23:43:00.755990Z",
"client_name": "My Project Admin Client",
"scope": "pangea:platform:account:read pangea:platform:account:manage",
"token_endpoint_auth_method": "client_secret_basic",
"redirect_uris": [],
"grant_types": [
"client_credentials"
],
"response_types": [
"token"
],
"client_token_expires_in": 3600,
"client_secret_id": "pce_3xwghy6y2pibrtagyottvfegxk2srvlz",
"client_secret": "pck_mz72lt...a3izf6",
"client_secret_expires_at": "2026-04-16T23:43:00.755028Z",
"client_secret_name": "My Project Admin Client Secret",
"client_secret_description": "Auto-created first client secret",
"owner_id": "pui_55ljz3kllliqrl43pxdgho4bk62qibzk",
"owner_username": "konstantin.lapine@pangea.cloud",
"creator_id": "psa_4lsuwezkztrhcfsidwltc4vt42idgtgn",
"client_class": "management",
"tenanted_by": "project"
}
The client secret, along with the client ID, is saved and managed in Vault. You can reference the secret by its client_secret_id
and use the Vault APIs to dynamically retrieve or rotate it.
Grant client additional access
You can use the /v1beta/oauth/clients/{client_id}/grant endpoint to extend a client's scope and set roles if this was not done during client registration. The following example demonstrates how to grant access to the Secure Audit Log configuration management APIs.
Parameters:
roles
- An array of role objects. The project management client created above does not accept additional roles, so the array can be left empty.scope
- A space-delimited list of additional scope values that the client can assign to its access tokens.
export PANGEA_CLIENT_ID="psa_qczxhxqgkc7lpvcmlfmx23pqwbyqnv7f"
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID/grant" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"roles": [],
"scope": "pangea:service:audit:config:manage"
}'
A successful grant request returns a 200
status code with an empty response body.
Revoke client access
You can partially or completely revoke a client's access using the /v1beta/oauth/clients/{client_id}/revoke endpoint. For example:
export PANGEA_CLIENT_ID="psa_qczxhxqgkc7lpvcmlfmx23pqwbyqnv7f"
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID/revoke" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"roles": [],
"scope": "pangea:service:audit:config:manage"
}'
A successful revocation returns a 200
status code with an empty response body.
Check client access
The client information returned from the registration endpoint or the /v1beta/oauth/clients/{clientId} endpoint includes the client’s API access, expressed via its scope.
export PANGEA_CLIENT_ID="psa_qczxhxqgkc7lpvcmlfmx23pqwbyqnv7f"
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID" \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN"
{
"client_id": "psa_qczxhxqgkc7lpvcmlfmx23pqwbyqnv7f",
"scope": "pangea:platform:account:read pangea:platform:account:manage",
...
}
To check the client’s permissions, you can retrieve its roles using the /v1beta/oauth/clients/{clientId}/roles endpoint.
curl --location "https://authorization.access.$PANGEA_DOMAIN/v1beta/oauth/clients/$PANGEA_CLIENT_ID/roles" \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN"
{
"roles": [
{
"type": "project",
"id": "ppi_vjmsx2q26gkqaxa2eit6karm2ccdbk7s",
"service": "",
"role": "admin"
}
],
"last": "MTox",
"count": 1
}
Platform Organization APIs
You can authorize requests to the Platform Organization APIs using an access token issued to a Management Client with the Org Admin role. The token must include the required scope and be passed as a bearer token.
These APIs allow you to retrieve organization details, update the organization name, list projects in the organization, and create or delete projects.
Get project list
For example, you can use an access token issued by an organization client to retrieve the list of projects in your organization.
export PANGEA_ACCESS_TOKEN="pts_mxui3w...wo7z56"
curl --location "https://api.console.$PANGEA_DOMAIN/v1beta/platform/project/list" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"org_id": "poi_qie5kaj5o3622tw4qj5qbyw77ship4bh"
}'
{
"result": {
"count": 31,
"next": null,
"previous": null,
"results": [
{
"id": "ppi_6bmgcbdovvre7k2t7y3iji3ewemy5c2s",
"name": "Service and Management APIs",
"org": "poi_qie5kaj5o3622tw4qj5qbyw77ship4bh",
"geo": "us",
"region": "us-west-2",
...
},
...
]
},
"status": "Success",
...
}
Platform Project APIs
You can use an organization-level or project-level Management Client to authorize requests to the Platform Project APIs by passing a bearer access token.
Learn more about managing project-level permissions in the Project API Credentials documentation.
export PANGEA_DOMAIN="aws.us.pangea.cloud"
export PANGEA_ACCESS_TOKEN="pts_mxui3w...wo7z56"
export PANGEA_PROJECT_ID="ppi_vjmsx2q26gkqaxa2eit6karm2ccdbk7s"
Get project details
For example, you can use it to retrieve the project details.
curl --location "https://api.console.$PANGEA_DOMAIN/v1beta/platform/project/get" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"id": "'"$PANGEA_PROJECT_ID"'"
}'
{
"summary": "Success",
"result": {
"id": "ppi_vjmsx2q26gkqaxa2eit6karm2ccdbk7s",
"name": "Service and Management APIs",
"org": "poi_qie5kaj5o3622tw4qj5qbyw77ship4bh",
"created_at": "2025-04-08T20:06:33.584508Z",
"updated_at": "2025-04-09T22:37:09.316433Z",
"geo": "us",
"region": "us-west-2",
"fqdn": "aws.us.pangea.cloud"
},
"status": "Success",
...
}
Update project
curl --location "https://api.console.$PANGEA_DOMAIN/v1beta/platform/project/update" \
--header 'Content-Type: application/json' \
--header "Authorization: Bearer $PANGEA_ACCESS_TOKEN" \
--data '{
"id": "'"$PANGEA_PROJECT_ID"'",
"name": "Service Gateway"
}'
{
"response_time": "2025-04-17T23:12:41.895Z",
"summary": "Success",
"result": {
"id": "ppi_vjmsx2q26gkqaxa2eit6karm2ccdbk7s",
"name": "Service Gateway",
"org": "poi_qie5kaj5o3622tw4qj5qbyw77ship4bh",
"created_at": "2025-04-08T20:06:33.584508Z",
"updated_at": "2025-04-17T23:12:41.666844Z",
"geo": "us",
"region": "us-west-2",
"fqdn": "aws.us.pangea.cloud"
},
"status": "Success",
...
}
Was this article helpful?